GitHub Actions: Dispatched & Scheduled Workflows with Inputs
I recently wrote a GitHub Actions workflow that I needed to be manually triggerable and scheduled via cron. My workflow had inputs. When the workflow is triggered by cron, I needed it to run with some default variables. When triggered manually, I wanted to allow the user to override some default values. It’s not immediately apparent how one should do this, but it is possible.
For example, I have the following GitHub Action workflow:
name: Print some text every 3rd minute
on:
schedule:
- cron: '1/3 * * * *'
jobs:
print_text:
runs-on: 'ubuntu-20.04'
steps:
-name: Print some text
run: echo "Periodically printing passages"
It prints out:
Periodically printing passages
You can imagine a workflow that executes automated deployments to some test environment. What if I wanted to allow users of my GitHub repo to trigger this manually and print custom text, with a default message available? You can accomplish this in the same workflow by using environmental variables.
name: Print some text every 3rd minute
on:
schedule:
- cron: '1/3 * * * *'
workflow_dispatch: # adding the workflow_dispatch so it can be triggered manually
inputs:
text_to_print:
description: 'What text do you want to print?'
required: true
default: 'Periodically printing passages'
jobs:
print_text:
runs-on: 'ubuntu-20.04'
steps:
- name: Set the variables
env:
DEFAULT_MESSAGE: 'Periodically printing passages' # here is the default message
run: echo "MESSAGE=${{ github.event.inputs.text_to_print || env.DEFAULT_MESSAGE }}" >> $GITHUB_ENV
- name: Print some text
run: echo "$MESSAGE" # prints the environmental variable, MESSAGE
I can trigger this in the Actions tag in GitHub:
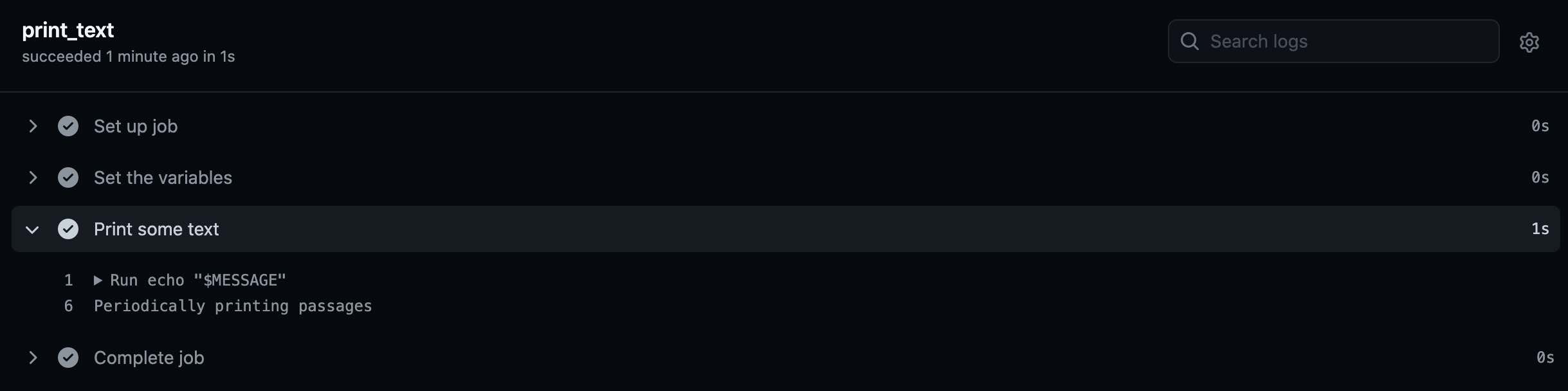
The key to getting this to work is:
echo "MESSAGE=${{ github.event.inputs.text_to_print || env.DEFAULT_MESSAGE }}" >> $GITHUB_ENV
The preceding line chooses to assign the defined variable to the variable MESSAGE
which is then injected into $GITHUB_ENV
. We can reference it in the Print some text
step.